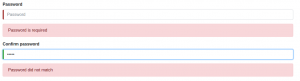
This blog represents code samples and related concepts on how to handle password confirmation logic in an Angular app (Angular 2/Angular 4). Note that the code samples make use of template-driven forms and uses [(ngModel)] for two-way data bindings.
Following are key aspects discussed in this blog:
- Template code for handling password confirmation logic
- Component code for handling password confirmation logic
- Signup model code
In this blog, it is considered that password confirmation logic is a key part of signup form. Thus, a model name Signup is used. However, the logic can also be used in update password form.
Following screenshot represents password confirmation UI related with the code given in this blog:
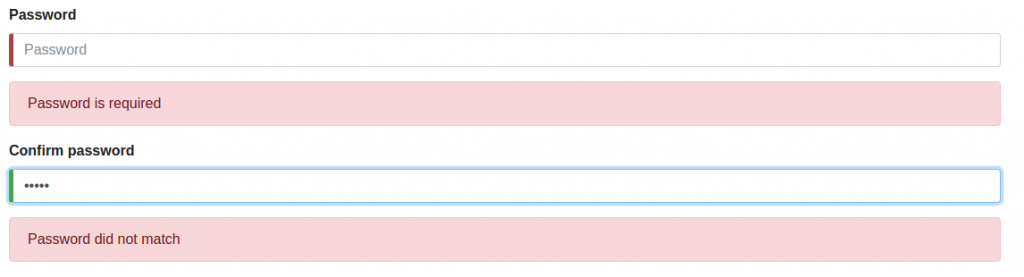
Figure 1. Password Confirmation Logic in Angular Form
Template Code for handling Password Confirmation Logic
<div class="form-group"> <label for="password"><strong>Password</strong></label> <input type="password" class="form-control" id="password" [(ngModel)]="signup.password" name="password" (blur)="confirmPassword()" required placeholder="Password" #pswd="ngModel"/> </div> <div [hidden]="pswd.valid || pswd.pristine" class="alert alert-danger">Password is required</div> <div class="form-group"> <label for="cnfpassword"><strong>Confirm password</strong></label> <input type="password" class="form-control" id="cnfpassword" [(ngModel)]="passwordConfirmationTxt" required (blur)="confirmPassword()" name="passwordConfirmationTxt"/> </div> <div [hidden]="!passwordConfirmationFailed" class="alert alert-danger">Password did not match</div>
Pay attention to some of the following in above code:
- Usage of (blur) event handler to invoke the method, confirmPassword
- Usage of template reference variable #pswd for tracking changes to required field, password
- Usage of passwordConfirmationFailed property to display the message “Password did not match” based on the whether its value is true or flag. Note the usage of [hidden]
Component Code for handling Password Confirmation Logic
export class SignupComponent { passwordConfirmationFailed = false; passwordConfirmationTxt = ''; signup = new Signup('', '', '', ''); confirmPassword() { if (this.signup.password === this.passwordConfirmationTxt) { this.passwordConfirmationFailed = false; } else { this.passwordConfirmationFailed = true; } } }
Signup Model Code
export class Signup { constructor ( public email: string, public name: string, public password: string, public country: string, ) { } }
In case you are developing web apps using Spring and Angular, check out my book, Building web apps with Spring 5 and Angular. Grab your ebook today and get started.
- Large Language Models (LLMs): Four Critical Modeling Stages - August 4, 2025
- Agentic Workflow Design Patterns Explained with Examples - August 3, 2025
- What is Data Strategy? - August 2, 2025
I found it very helpful. However the differences are not too understandable for me