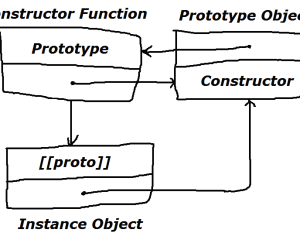
This article represents tips and code samples on How to create and instantiate a Class in NodeJS. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos.
Following are the key points described later in this article:
- How to Create a Class?
- How to Instantiate a Class?
How to Create a Class?
// This is a Constructor function taking age and passport
// as the paramaters
function Person(age, passport) {
this.age = age;
this.passport = passport;
}
// Sets the age
//
Person.prototype.setAge = function(age) {
this.age = age;
};
// Checks whether the person is Adult based on the age
//
Person.prototype.isAdult = function() {
return this.age >= 18? true: false;
};
// Checks whether the person can have bank accounts
// based on whether he/she is an adult
//
Person.prototype.canHaveBankAccounts = function() {
return this.isAdult()?true:false;
};
// Sets the passport status of the person
//
Person.prototype.passportStatus = function(status) {
this.passport = status;
};
// Checks whether the person has the passport
//
Person.prototype.hasPassport = function() {
return this.passport;
};
// Sets the Person object to module.exports
//
module.exports = Person;
How to Instantiate a Class?
Following code also demonstrates the following:
- Check the parameters passed to “node” command: The function used is “process.argv”. The first element is “node” command. The second element at index 1 is file name. Third element is first parameter passed to the “node” command.
- Exit the execution: The function used is “process.exit()”.
var Person = require("./Person.js");
// Checks whether minimum of 3 parameters have been entered
//
if(process.argv.length <= 2) {
console.log("You must pass the age of the person.");
// Exists
process.exit();
}
// Creates the person using the constructor function
//
var person = new Person(1, false);
console.log("Person is an adult is: " + person.isAdult());
console.log("Person can have bank account is: " + person.canHaveBankAccounts());
// Sets the age of the person
//
person.setAge(process.argv[2]);
console.log("Person is an adult is: " + person.isAdult());
console.log("Person can have bank account is: " + person.canHaveBankAccounts());
Latest posts by Ajitesh Kumar (see all)
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me