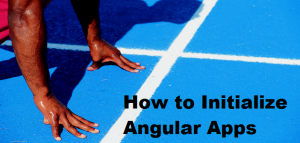
Following are the key points described later in this article:
- Automatic Initialization
- Manual Initialization
Automatic Initialization
All that is needed for automatic initialization (as of current AngularJS version) is to define “ng-app” on an element and you should be all set. Take a look at following code sample. Pay attention to some of the following:
- ng-app=”HelloApp” defined on div element
- ng-controller=”HelloCtrl” defined on the same element. It could as well be defined within the inner elements.
<!DOCTYPE html>
<html>
<head>
<title>Hello Angular</title>
<link rel="stylesheet" type="text/css" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css">
</head>
<body>
<div ng-app="HelloApp" ng-controller="HelloCtrl">
<h1>Hello, {{name}}</h1>
<form>
<input type="text" ng-model="name" name="name">
</form>
</div>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.3/angular.min.js"></script>
<script type="text/javascript">
angular.module('HelloApp', [])
.controller('HelloCtrl', ['$scope', function($scope){
$scope.name = "Calvin";
}])
</script>
</body>
</html>
As per the description on this page, Angular initializes automatically upon DOMContentLoaded event or when the angular.js script is evaluated if at that time document.readyState is set to ‘complete’. At this point, Angular looks for the ng-app directive which designates your application root. Read further on the given page.
Manual Initialization
One could also initialize the angular app manually. This seems to be suited more for existing/legacy web pages where you would want to initialize angular app for a particular set of “views” or, initialize the app conditionally. Following is the code sample. Pay attention to the following code snippet which is used to instantiate the angular app manually. angular.bootstrap(document, [‘HelloApp’]);. Read further on this page.
<!DOCTYPE html>
<html>
<head>
<title>Hello Angular</title>
<link rel="stylesheet" type="text/css" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css">
</head>
<body>
<div ng-controller="HelloCtrl">
<h1>Hello, {{name}}</h1>
<form>
<input type="text" ng-model="name" name="name">
</form>
</div>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.3/angular.min.js"></script>
<script type="text/javascript">
angular.module('HelloApp', [])
.controller('HelloCtrl', ['$scope', function($scope){
$scope.name = "Calvin";
}])
angular.bootstrap(document, ['HelloApp']);
</script>
</body>
</html>
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply