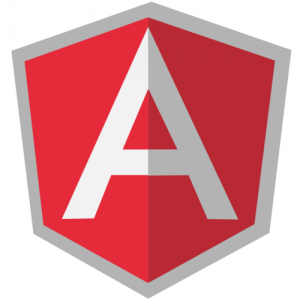
This article represents high level concepts and code examples on how to create a custom directive. For detailed documentation, one could access AngularJS page on custom directive. You could check out live demo of the code example in this article on http://hello-angularjs.appspot.com/angularjs-directives-hello-world
Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos.
Following are the key points described later in this article:
- Key aspects of Directive
- Code Examples – Directives Hello World
Key Aspects of Directive
The directive discussed in the blog is following:
<hello name="Calvin Hobbes"></hello>
Following are some of the key aspects:
- Define directive. In this example, directive is defined as part of separate module and later, the module in included as a dependency when instantiating another angular app in the page.
- Define templates. Template is the file (HTML code representing one or more DOM elements) that results in attaching one or more DOM elements to original DOM as part of compiling/linking the directive
Code Examples – Directives Hello World
Following are key aspects of working with the code given below:
- Directive code:Create js folder. Create an app.js file within js folder and paste the directive code given below
- Template code:Create templates folder. Create hello.html template file within templates folder and paste the template code given below.
- HTML page code:In the root folder that consists of above two folders (js & templates), create index.html and paste the HTML page code given below.
Directive Code
Pay attention to the usage of some of the key aspects of directive used in the code below:
Pay attention to the usage of some of the key aspects of directive used in the code below:
- restrict keyword which restricts the usage of directive as only “element”. Other possible usages are Attribute (A), Class (C) and Comment (M)
- scope is isolated meaning, scope is not inherited from the parent. Pay the attention of usage of ‘@’. @ binds a local/directive scope property to the evaluated value of the DOM attribute. If one use name=”Calvin Hobbes”, the value of DOM attribute “name” is simply the string “Calvin Hobbes”. Other possibility is usage of ‘=’. = binds a local/directive scope property to a parent scope property. So with =, one use the parent model/scope property name as the value of the DOM attribute.
- templateUrl is used to specify the location of template file
- Directive Definition Object (DDO) is returned. Note that it does not have any link function because it was not required to execute the directive. It also does not consist of controller function as well. Controller function is used to define one or more APIs that could be called from other directives.
angular.module('HelloModule', [])
.directive( 'hello', function() {
return {
restrict: "E",
scope:{
name: '@'
},
templateUrl: 'templates/hello.html'
}
})
Template Code
<div>
<input type="text" ng-model="name" value="{{name}}"/>
</div>
<div>
<h3>Hello {{ name }}!</h3>
</div>
HTML Page Code
Pay attention to some of the following:
Pay attention to some of the following:
- For directive to work, HelloModule is included as the HelloApp is instantiated
- Usage of directive “hello” with name attribute.
<!DOCTYPE html>
<html ng-app="HelloApp">
<head>
<title>AngularJS - Custom Directive Hello World - Code Example</title>
<link rel="stylesheet" type="text/css" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css">
</head>
<body ng-controller="HelloCtrl" class="container">
<div class="page-header"><h2>AngularJS - Custom Directive Hello World - Code Example</h2></div>
<hello name="Calvin Hobbes"></hello>
<bye></bye>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<script type="text/javascript" src="js/app.js"></script>
<script type="text/javascript">
angular.module('HelloApp', ['HelloModule'])
.controller('HelloCtrl', ['$scope', function($scope){
}])
</script>
</body>
</html>
…
[adsenseyu1]
Latest posts by Ajitesh Kumar (see all)
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me