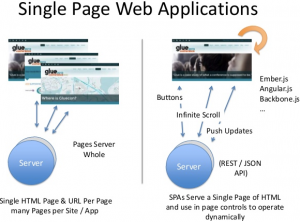
- What is Single-Page application?
- Routing in AngularJS – Key for SPA
- Code Sample – Single Page App
- Code Sample – Routing
Some of the key AngularJS concepts that got demonstrated in this article and the related demo page are following:
- Routing
- Directives
- Templating
- Two-way data binding
What is Single-Page application (SPA)?
As defined on Wikipedia page, “a single-page application (SPA), also known as single-page interface (SPI), is a web application or web site that fits on a single web page with the goal of providing a more fluid user experience akin to a desktop application. In an SPA, either all necessary code “HTML, JavaScript, and CSS” is retrieved with a single page load, or the appropriate resources are dynamically loaded and added to the page as necessary, usually in response to user actions. The page does not reload at any point in the process, nor does control transfer to another page…”.
In my early days of programming, I developed an entire website, funpiper.com, based on the concept of single page application, however, with great amount of complexity. Most of the pages on the website was loaded with home.php with pagetype passed as a GET parameter. This pagetype consisted of PAGE identifier which was used to load appropriate view with header and footer code getting loaded with home.php. Some of the key issues were UI code (routing) written in server pages along with maintenance nightmare. Now that I played with AngularJS, I could really appreciate the power of Routing concept in AngularJS and how easily one could create & manage single-page application using the same.
Following are key design steps in creating SPA with Angular:
- Identify single page design elements including different views
- Identify one or more controllers that would be used with these views
- Glue views and controllers together with Angular routing
Routing in AngularJS – Key to SPA
Routing functionality provided by Angular helps you to create a single page app where multiple views could be loaded in the single page based on the URL parameter. Different views are accessed using following URL format:
- http://<some.website.com>/singlepage#/view1
- http://<some.website.com>/singlepage#/view2
- http://<some.website.com>/singlepage#/view3
The routing functionality is provided by Angular ngRoute module, which is distributed separately from the core Angular framework. Take a look at the code below under sinple page app to see different library (angular-route.js) for ngRoute module.
Application routes in Angular are declared via the $routeProvider, which is the provider of the $route service. This service makes it easy to wire together controllers, view templates, and the current URL location in the browser. Access greater details on this page (https://docs.angularjs.org/tutorial/step_07).
Code Sample – Single Page App
Following is the code sample for most trivial SPA. Pay attention to some of the following:
- Directive ng-app=”helloApp”: Defines the HTML page as angular module namely, helloApp
- Inclusion of two Angular javascript files; They are loaded from Google hosted libraries. Notice different library (angular-route.js) needed for loading ngRoute module which makes the routing possible.
- Directive ng-view which is used to load different views based on routing configuration (URL)
- The page has its own URL. The code below is demonstrated at http://hello-angularjs.appspot.com/spa
<html ng-app="helloApp"> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.18/angular.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.18/angular-route.js"></script> <script src="myangular.js"></script> </head> <body> <div ng-view=""></div> </body> </html>
Code Sample – Routing
Following code represents the Angular module definition along with routing configuration. Pay attention to some of the following:
- First line creates an angular module namely “helloApp” which depends on the module, “ngRoute”.
- helloApp module is configured with a routeProvider which defines different routes such as ‘/searchtable’ which is displayed with view such as ‘views/searchtable.html’ that is related with controller, ‘CompanyCtrl’.
- The views could be accessed using following URL format if the SPA URL is http://hello-angularjs.appspot.com/spa
- http://hello-angularjs.appspot.com/spa#searchtable
- http://hello-angularjs.appspot.com/spa#sorttablecolumn
var helloApp = angular.module("helloApp", ['ngRoute']); helloApp.config(function($routeProvider){ $routeProvider .when( '/searchtable', { controller: 'CompanyCtrl', templateUrl: 'views/searchtable.html' } ) .when('/sorttablecolumn', { controller: 'CompanyCtrl', templateUrl: 'views/sorttablecolumn.html' }) .otherwise( { redirectTo: '/searchtable' } ); });
[adsenseyu1]
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me