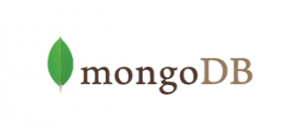
Key Concepts
Simply speaking, MongoDB is a very popular NoSQL database with document-oriented storage. If you are a SQL developer and having challenges understanding document-oriented database, check out this page on mapping between SQL to MongoDB mapping. For a detailed introduction on MondoDB, check out this introduction page.
Following are some of the key terminologies:
- Database
- Collections
- Document
- Field
- Primary key (_id)
- index
JSON-styled Documents: The most important concept is document-oriented storage, and the documents are JSON-styled. Thus, one would require to learn JSON very well to do well with MongoDB as JSON-styled document is at the heart of it.
As like MySQL server and client, MongoDB also has a server and client. Following are the commands:
mongod: Start the server
mongo: Start the client
Document:
Following is a sample document in the collection, “users”:
{
_id: ObjectId("509a8fb2f3f4658bd2f783a0"),
firstname: "Chris",
lastname: "Johnson",
age: 25,
email: "chris.johnson@gmail.com"
}
Collection:
Simply speaking, Collection is a set of documents.
Key Commands
Before I list down some, I must say that you may see a detailed query samples in comparison with traditional SQL statements on this page.
- show dbs: Shows all the databases
- use <dbname>: Use the database; Create the database if not present in the database list
- show collections: Shows all the collections in any database
CRUD: Create A Collection Explicity
db.createCollection( "<collectionname>" )
CRUD: Create A Document
db..insert( ): Create the collection if does not exist and insert the document OR, Insert the document in the existing collection having name as . Following is the sample command:
//Insert a new document in the collection users
db.users.insert(
{
firstname: "Bill",
lastname: "Sherwood",
age: 32,
email: "bill1994@hotmail.com"
}
)
// Insert multiple documents or array of documents
db.users.insert(
[
{ firstname: "Bill", lastname: "Sherwood", age: 32,email: "bill1994@hotmail.com"},
{ firstname: "Chris", lastname: "Johnson", age: 28,email: "chris123@gmail.com"},
]
)
CRUD: Select Documents
//Shows all the documents within a collection.
db.<collectionname>.find()
CRUD: Update Documents
//Updates multiple documents set firstname = "William" whose age = 35
db.users.update(
{ age: 35 },
{ $set: { firstname: "William" } ,
{ multi: true }
)
CRUD: Delete Document
// Removes the document with age=35
db.users.remove(
{ age: 35 }
)
// Removes all the document
db.users.remove( {} )
As mentioned earlier, if you want to quickly learn these commands with equivalent MySQL commands in the sight, do bookmark the following link: http://docs.mongodb.org/manual/reference/sql-comparison/
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
- How to Setup MEAN App with LangChain.js - February 9, 2025
- Build AI Chatbots for SAAS Using LLMs, RAG, Multi-Agent Frameworks - February 8, 2025
Good intro. I did a free course on MongoDB University last year and found it very good. I think they started a spell this week again, check https://university.mongodb.com/. I highly recommend the M101J course, it’s free!