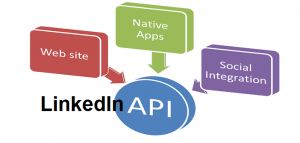
This article represents code samples and changes that need to be done in original Linkedin-J framework (open-source) to get company updates of different types such as following:
- Job Posting
- Status Updates
- New Product
Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos.
Code Samples & Steps to Get Company Updates
Following files need to be changed along with mentioned changes:
- LinkedinApiUrls.properties: Following code needs to be added under APIs mentioned under companies:
com.google.code.linkedinapi.client.getCompanyStatusUpdates=http://api.linkedin.com/v1/companies/{id}/updates{queryParameters}
- LinkedInApiUrls.java: Following code needs to be added:
public static final String GET_COMPANY_STATUS_UPDATES = linkedInApiUrls.getProperty("com.google.code.linkedinapi.client.getCompanyStatusUpdates");
- CompaniesApiClient.java: Following APIs need to be added:
/** * Gets the company by id. * * @param id the id * * @return the company by id */ public Updates getCompanyStatusUpdates(String id); /** * Get comanpy updates by companyid and event type * @param id * @param eventType * @return */ public Updates getCompanyStatusUpdates(String id, String eventType ); /** * Get company updates by companyid, event type, start index, count * @param id * @param eventType * @param start * @param count * @return */ public Updates getCompanyStatusUpdates(String id, String eventType, int start, int count);
- BaseLinkedInApiClient.java: Following implementations need to be added:
@Override public Updates getCompanyStatusUpdates(String id) { assertNotNullOrEmpty("id", id); LinkedInApiUrlBuilder builder = createLinkedInApiUrlBuilder(LinkedInApiUrls.GET_COMPANY_STATUS_UPDATES); String apiUrl = builder.withEmptyField(ParameterNames.FIELD_SELECTORS).withField(ParameterNames.ID, id).buildUrl(); return readResponse(Updates.class, callApiMethod(apiUrl)); } @Override public Updates getCompanyStatusUpdates(String id, String eventType) { assertNotNullOrEmpty("id", id); assertNotNullOrEmpty("eventtype", eventtype); LinkedInApiUrlBuilder builder = createLinkedInApiUrlBuilder(LinkedInApiUrls.GET_COMPANY_STATUS_UPDATES); String apiUrl = builder.withEmptyField(ParameterNames.FIELD_SELECTORS) .withField(ParameterNames.ID, id) .withParameter(ParameterNames.EVENT_TYPE, eventType).buildUrl(); return readResponse(Updates.class, callApiMethod(apiUrl)); } @Override public Updates getCompanyStatusUpdates(String id, String eventType, int start, int count) { assertNotNullOrEmpty("id", id); LinkedInApiUrlBuilder builder = createLinkedInApiUrlBuilder(LinkedInApiUrls.GET_COMPANY_STATUS_UPDATES); String apiUrl = builder.withEmptyField(ParameterNames.FIELD_SELECTORS) .withField(ParameterNames.ID, id) .withParameter(ParameterNames.EVENT_TYPE, eventType) .withParameter(ParameterNames.START, String.valueOf(start)) .withParameter(ParameterNames.COUNT, String.valueOf(count)) .buildUrl(); return readResponse(Updates.class, callApiMethod(apiUrl)); }
Once above files have been modified, you are all set to access the company status updates using following code:
public static void main(String[] args) {
String consumerKeyValue = "your-consumer-key";
String consumerSecretValue = "your-consumer-secret";
String accessTokenValue = "use-your-access-token-value";
String tokenSecretValue = "use-your-token-secret-value";
final LinkedInApiClientFactory factory = LinkedInApiClientFactory.newInstance(consumerKeyValue, consumerSecretValue);
final LinkedInApiClient client = factory.createLinkedInApiClient(accessTokenValue, tokenSecretValue);
Updates updates = client.getCompanyStatusUpdates( "1441", "status-update", 10, 10 );
List ulist = updates.getUpdateList();
if( ulist != null ) {
Iterator uiter = ulist.iterator();
while( uiter.hasNext() ) {
Update update = uiter.next();
CompanyStatusUpdate csu = update.getUpdateContent().getCompanyStatusUpdate();
if( csu != null ) {
System.out.println( "Status Updates: " + cssu.getShare().getComment() );
}
}
}
}
Latest posts by Ajitesh Kumar (see all)
- Agentic Reasoning Design Patterns in AI: Examples - October 18, 2024
- LLMs for Adaptive Learning & Personalized Education - October 8, 2024
- Sparse Mixture of Experts (MoE) Models: Examples - October 6, 2024
I found it very helpful. However the differences are not too understandable for me