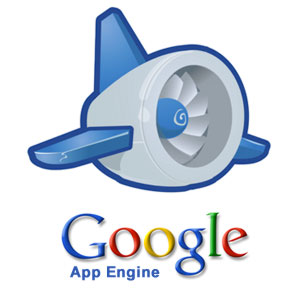
Following are code samples on Google App Engine Datastore Query and how to get entities by id and based on filters.
Get Entity By Id
Pay attention to the code “datastore.get(KeyFactory.createKey( “savedreport”, reportId). “savedreport” is the name of entity.
DatastoreService datastore = DatastoreServiceFactory.getDatastoreService();
Entity entity = null;
try {
entity = datastore.get(KeyFactory.createKey("savedreport", reportId));
} catch(EntityNotFoundException e) {
e.printStackTrace();
}
Get Entity By One Filter
Pay attention to “setFilter” method
Filter createdByFilter = new FilterPredicate("created_by", FilterOperator.EQUAL, userId );
Query query = new Query("sqm").setFilter( createdByFilter );
DatastoreService datastore = DatastoreServiceFactory.getDatastoreService();
List entities = datastore.prepare(query).asList( FetchOptions.Builder.withLimit( count ) );
Get Entity By Multiple Filter
Pay attention to usage of multiple FilterPredicate methods and CompositeFilterOperator and setFilter method called on Query.
Filter dateMinFilter = new FilterPredicate("sprint_enddate", FilterOperator.GREATER_THAN_OR_EQUAL, beginDate );
Filter dateMaxFilter = new FilterPredicate("sprint_enddate", FilterOperator.LESS_THAN_OR_EQUAL, endDate );
Filter nameFilter = new FilterPredicate("project_id", FilterOperator.EQUAL, projectId );
Filter createdByFilter = new FilterPredicate("created_by", FilterOperator.EQUAL, createdBy );
Filter rangeFilter = CompositeFilterOperator.and( nameFilter, createdByFilter, dateMinFilter, dateMaxFilter );
Query query = new Query("sqm").setFilter( rangeFilter );
DatastoreService datastore = DatastoreServiceFactory.getDatastoreService();
List entities = datastore.prepare(query).asList( FetchOptions.Builder.withLimit( count ) );
Latest posts by Ajitesh Kumar (see all)
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
- How to Setup MEAN App with LangChain.js - February 9, 2025
I found it very helpful. However the differences are not too understandable for me