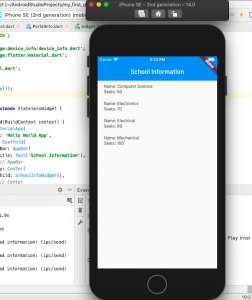
In this post, we will understand the concepts in relation to creating a Dart object from a JSON object and how to use these DART objects in Flutter app. We will build an app and run the code shown in this post.
Here is an example JSON object which we will work with, in order to create a custom Dart object called as School. The JSON represents information about a school including name, address, departments. You may note that the JSON object is primarily of type Map<String, dynamic> where key is String and value is of dynamic type. The key, name, is of type <String, String>, the key, address, is of type <String, Object> and the key, departments is of type <String, List<Object>>
{
"name": "IIT Kharagpur",
"address": {"city": "Kharagpur", "state": "West Bengal"},
"departments": [
{"name": "Computer Science",
"seats": 50
},
{"name": "Electronics",
"seats": 70
},
{"name": "Electrical",
"seats": 80
},
{"name": "Mechanical",
"seats": 100
},
]
}
In order to read above object, we will need to create a DART representation of School. School class will be composed of an Address and List of Department objects. Take a look at the following code. It comprises of three classes.
- School class which comprises of three member variables such as name, address and list of departments
- Address class
- Department class
Each of the classes have static or factory method to create the respective DART object from JSON object
// School Object
class School {
String name;
Address address;
List<Department> departments;
School({
this.name,
this.address,
this.departments
});
static fromJson(Map<String, dynamic> parsedJson){
return School(
name: parsedJson['name'],
address : Address.fromJson(parsedJson['address']),
departments : Department.listFromJson(parsedJson['departments'])
);
}
}
//
// Department object
//
class Department {
String name;
int seats;
Department({
this.name,
this.seats
});
static listFromJson(List<Map<String, dynamic>> list) {
List<Department> departments = [];
for (var value in list) {
departments.add(Department.fromJson(value));
}
return departments;
}
static fromJson(Map<String, dynamic> parsedJson){
return Department(
name: parsedJson["name"],
seats: parsedJson["seats"]
);
}
}
//
// Address object
//
class Address {
String city;
String state;
Address({
this.city,
this.state
});
static fromJson(Map<String, dynamic> parsedJson){
return Address(
city: parsedJson["city"],
state: parsedJson["state"]
);
}
}
Once we have the Dart representation of objects ready, we will see how to read and display the JSON object on Flutter app. A stateless widget, SchoolInfoWidget is defined and the build method is used to build the widget / element tree as shown in the diagram later. Pay attention to the code School schoolInfo = School.fromJson(_school) which is passed the JSON object and returns the School DART object.
class SchoolInfoWidget extends StatelessWidget {
Map<String, dynamic> _school = {
"name": "IIT Kharagpur",
"address": {
"city": "Kharagpur",
"state": "West Bengal"
},
"departments": [
{"name": "Computer Science",
"seats": 50
},
{"name": "Electronics",
"seats": 70
},
{"name": "Electrical",
"seats": 80
},
{"name": "Mechanical",
"seats": 100
},
]
};
@override
Widget build(BuildContext context) {
School schoolInfo = School.fromJson(_school);
return ListView(
children: List.generate(schoolInfo.departments.length,(index){
return Container(
padding: const EdgeInsets.fromLTRB(20.0, 10.0, 10.0, 10.0),
child: Text("Name: " + schoolInfo.departments[index].name.toString() +"\n" "Seats: " + schoolInfo.departments[index].seats.toString())
);
})
);
}
}
Finally, we will load the SchoolInfoWidget in the Flutter app using the following code:
import 'dart:io';
import 'package:device_info/device_info.dart';
import 'package:flutter/material.dart';
import 'School.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Hello World App',
home: Scaffold(
appBar: AppBar(
title: Text('School Information'),
),
body: Center(
child: SchoolInfoWidget(),
),
));
}
}
And, running above app will look like the following in the mobile device.
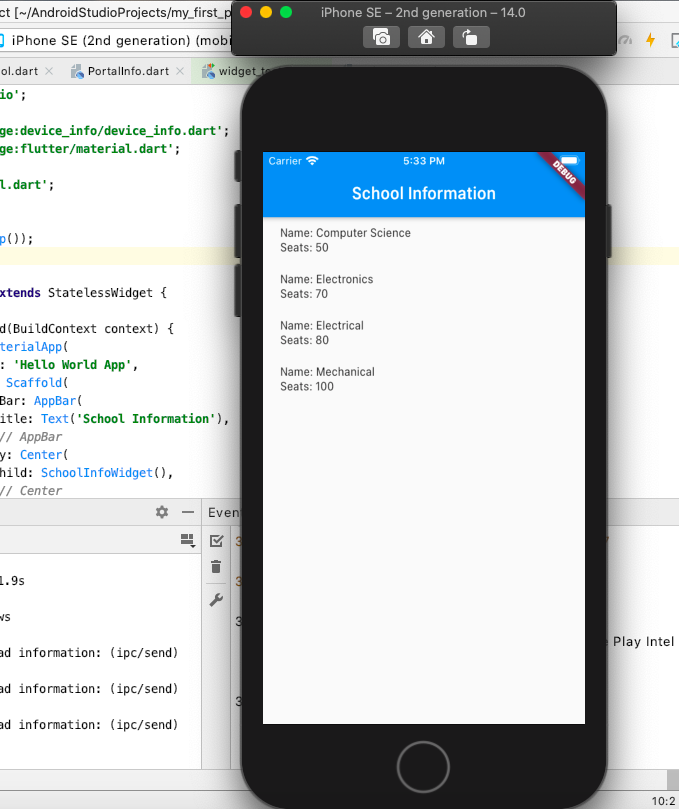
The code given in this post will be very useful in reading JSON from REST API response as a set of DART objects.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply