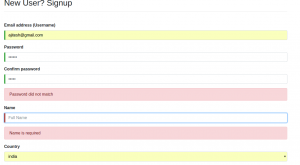
This blog represents code samples and related concepts which can be used to create a Signup form in Angular (Angular 2/Angular 4).In order to create signup form, following code is required to be written:
- Model class for Signup
- Template (UI) code for Signup
- Component code for handling Signup Form
- Style code for visually handling input validation
- Module code that includes the SignupComponent
This is how the screenshot of the Signup form looks like:
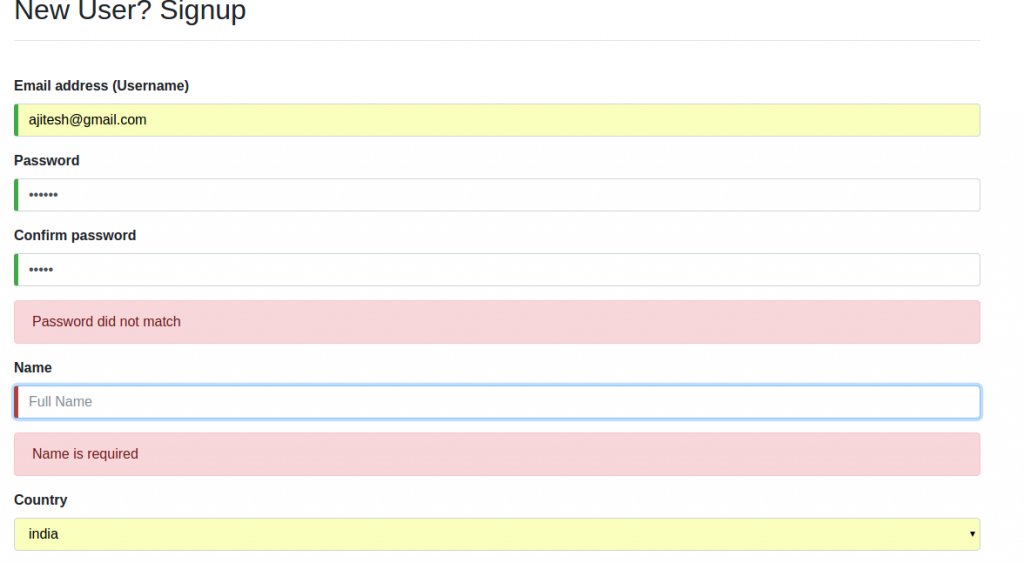
Figure 1. Angular Signup Form Template
Following are different files which may need to be created to manage signup module:
- signup.ts (Model class)
- signup.component.ts (Component)
- signup.component.html (Template)
- signup.component.css (CSS styles that are used in template)
- app.module.ts or signup.module.ts (in case, signup is a separate feature module)
Model Class for Signup
Save the code given below in a file namely, signup.ts
export class Signup { constructor ( public email: string, public name: string, public password: string, public country: string, ) { } }
Angular Template (UI) code for Signup Form
Save the following in a file, namely, signup.coomponent.html
<div style="margin-top: 20px"> <h2>New User? Signup</h2> <hr/> </div> <div style="margin-top: 30pt"> <form (ngSubmit)="onSubmit()" #signupForm="ngForm"> <div class="form-group"> <label for="emailField"><strong>Email address</strong></label> <input type="email" class="form-control" id="emailField" [(ngModel)]="signup.email" required placeholder="name@example.com" name="uEmail" #email="ngModel"/> </div> <div [hidden]="email.valid || email.pristine" class="alert alert-danger">Email address is required</div> <div class="form-group"> <label for="password"><strong>Password</strong></label> <input type="password" class="form-control" id="password" [(ngModel)]="signup.password" name="password" (blur)="confirmPassword()" required placeholder="Password" #pswd="ngModel"/> </div> <div [hidden]="pswd.valid || pswd.pristine" class="alert alert-danger">Password is required</div> <div class="form-group"> <label for="cnfpassword"><strong>Confirm Password</strong></label> <input type="password" class="form-control" id="cnfpassword" [(ngModel)]="passwordConfirmationTxt" (blur)="confirmPassword()" name="passwordConfirmationTxt"/> </div> <div [hidden]="!passwordConfirmationFailed" class="alert alert-danger">Password did not match</div> <div class="form-group"> <label for="fullname"><strong>Name</strong></label> <input class="form-control" id="fullname" [(ngModel)]="signup.name" placeholder="Full Name" required name="uName" #fullname="ngModel"/> </div> <div [hidden]="fullname.valid || fullname.pristine" class="alert alert-danger">Name is required</div> <div class="form-group"> <label for="country"><strong>Country</strong></label> <select class="form-control" id="country" required> <option *ngFor="let country of countries" [value]="country">{{country}}</option> </select> </div> <button type="submit" class="btn btn-success" [disabled]="!signupForm.form.valid">Submit</button> </form> </div>
Angular Component (class) code for handling Signup Form
Save the following code in a file such as signup.component.ts.
import { Component } from '@angular/core'; import {Signup} from './signup'; @Component({ selector: 'app-root', templateUrl: './signup.component.html', styleUrls: ['./signup.component.css'] }) export class SignupComponent { title = ''; passwordConfirmationFailed = false; passwordConfirmationTxt = ''; signup = new Signup('', '', '', ''); countries = ['india', 'canada', 'us']; confirmPassword() { if (this.signup.password === this.passwordConfirmationTxt) { this.passwordConfirmationFailed = false; } else { this.passwordConfirmationFailed = true; } } onSubmit() { console.log('Name: ' + this.signup.name + ', Email: ' + this.signup.email + ', Password: ' + this.signup.password ); } }
Styles for visually handling Input Validation
Save the code given below in file such as signup.component.css which can be found in the same folder. Note that the styles file is referenced in the component code with the property such as styleUrls. Refer the code given above.
.ng-valid[required], .ng-valid.required { border-left: 5px solid #42A948; /* green */ } .ng-invalid:not(form) { border-left: 5px solid #a94442; /* red */ }
Module code for including Signup Component
Pay attention to some of the following in the code given below:
- FormsModule is declared in imports array
- SignupComponent is declared in declarations array
Save the code in a file namely, app.module.ts or signup.module.ts (if Signup is a separate feature module)
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { SignupComponent } from './signup.component'; import {FormsModule} from '@angular/forms'; @NgModule({ declarations: [ SignupComponent ], imports: [ BrowserModule, FormsModule ], providers: [], bootstrap: [SignupComponent] }) export class AppModule { }
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me