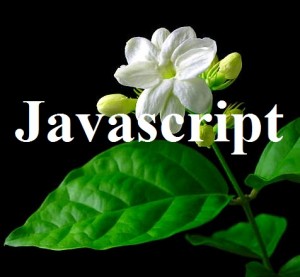
The article lists down some of the unit tests samples for testing Javascript code. The unit tests in this article tests the javascript code presented in this article, “What are Objects in Javascript?”.
Before presenting code samples, lets try and understand what is Jasmine?
As on the Jasmine website, it is defined as a behavior-driven development framework for testing JavaScript code. It does not depend on any other JavaScript frameworks. It does not require a DOM. And it has a clean, obvious syntax so that you can easily write tests.
By what I learnt so far, I could vouch for the statement “And it has a clean, obvious syntax so that you can easily write tests“. It was very easy for me to understand and write unit tests in no time. I must admit that I am familiar with the notion of unit tests and written several unit tests in Java in my past experience. Also, it would be good to have a knowledge on BDD (Behavior driven development) to get a good hang on Jasmine unit testing framework.
The key to Jasmine unit testing is following:
- Test suite defined using “describe”. The syntax goes like following: describe( “some name”, function() { …code goes here… } );
- Test cases defined using “it”. The syntax goes like following: it( “some text”, function() {…code goes here…} );
Pay attention to the naming scheme of test suite and test spec. The names are based on BDD naming standard (usage of should). For example, in case of test suite, School, the names of “describe” and “it” when read together forms a sentence with appropriate meaning.
- School admission status should be false by default
- School admission status should change after set
#Unit Test Code Sample 1
The code below tests the object, School whose code is presented in following page: “What are Objects in Javascript?”
describe( "School", function() {
it( "admission status should be false by default", function() {
expect( School.admissionOpen ).toBe( false );
});
it( "admission status should change after set", function() {
School.setAdmissionStatus( true );
expect( School.isAdmissionOpen() ).toBe( true );
});
});
#Unit Test Code Sample 2
The code below tests the object, College, whose code is presented in following page: “What are Objects in Javascript?”
describe( "College", function(){
it( "admission status is false by default", function(){
expect(College.admissionStatus).toBe( false );
});
it("admission status to true after set as true", function(){
College.setAdmissionStatus( true );
expect( College.isAdmissionOpen() ).toBe( true );
});
});
#Unit Test Code Sample 3
The code below tests the object, EducationalInstitution, whose code is presented in following page: “What are Objects in Javascript?”
Following code made use of beforeEach function which defines the objects, davPublicSchool and iitKharagpur before each test is executed.
describe( "EducationalInstitution", function(){
var davPublicSchool;
var iitKharagpur;
beforeEach(function() {
davPublicSchool = new EducationalInstitution( "Dav Public School", 650 );
iitKharagpur = new EducationalInstitution( "IIT Kharagpur", 3500 );
});
it("constructor should create objects", function(){
expect( davPublicSchool ).not.toBeNull();
expect( iitKharagpur ).not.toBeNull();
});
it("constructor should create different objects", function(){
expect( davPublicSchool.name ).not.toMatch( iitKharagpur.name );
expect( davPublicSchool.strength ).not.toMatch( iitKharagpur.strength );
});
});
SpecRunner Code Example
The above tests could be run with following specRunner code placed inside Jasmine installation folder. Download the Jasmine from this page.
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Jasmine Spec Runner v2.0.0</title>
<link rel="shortcut icon" type="image/png" href="lib/jasmine-2.0.0/jasmine_favicon.png">
<link rel="stylesheet" type="text/css" href="lib/jasmine-2.0.0/jasmine.css">
<script type="text/javascript" src="lib/jasmine-2.0.0/jasmine.js"></script>
<script type="text/javascript" src="lib/jasmine-2.0.0/jasmine-html.js"></script>
<script type="text/javascript" src="lib/jasmine-2.0.0/boot.js"></script>
<!-- include source files here... -->
<script type="text/javascript" src="src/School.js"></script>
<!-- include spec files here... -->
<script type="text/javascript" src="spec/SchoolSpec.js"></script>
</head>
<body>
</body>
</html>
…
[adsenseyu1]
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me